Controlling Response Length and Randomness in Google App Script with AI Language Models
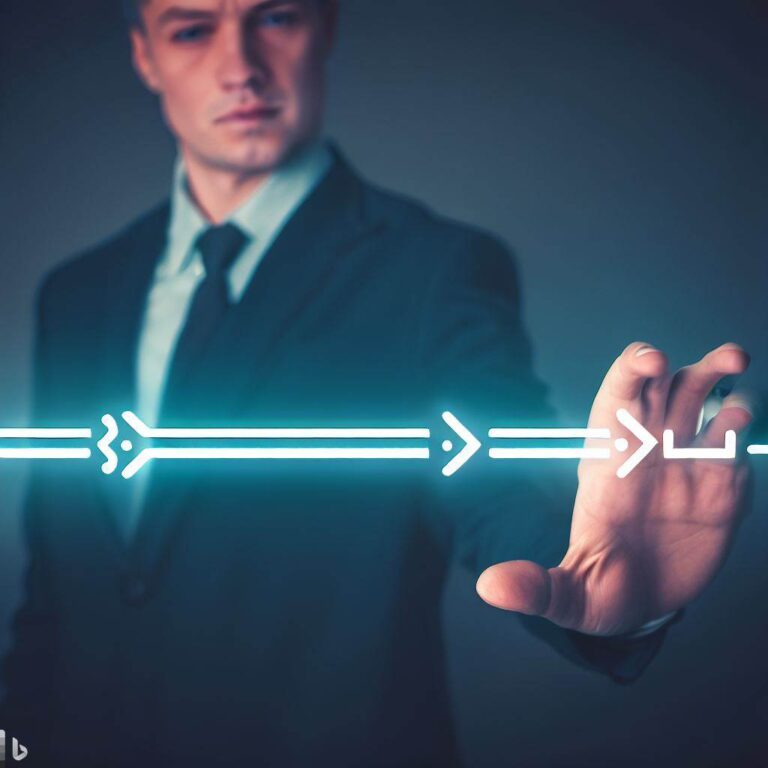
Throughout my website, following the links to any of my affiliates and making a purchase will help support my efforts to provide you great content! My current affiliate partners include ZimmWriter, LinkWhisper, Bluehost, Cloudways, Crocoblock, RankMath Pro, Parallels for Mac, AppSumo, and NeuronWriter (Lifetime Deal on AppSumo).
For tutorials on how to use these, check out my YouTube Channel!
When using AI language models like GPT-3.5 Turbo and GPT-4 in your Google App Script applications, controlling the response length and randomness can help you achieve the desired output for your specific use case. This blog post will explore methods to fine-tune the generated content by adjusting parameters such as max_tokens
and temperature
when interacting with AI language models.
By the end of this post, you will learn how to control response length and randomness in Google App Script applications, allowing you to create more targeted and efficient AI-driven content.
Controlling Response Length with Max Tokens
Language models like GPT-3.5 Turbo and GPT-4 generate content based on tokens, which represent chunks of text like words or word pieces. By setting the max_tokens
parameter, you can control the response length of the generated content.
In Google App Script, you can set the max_tokens
parameter when making an API call to the AI language model. Here’s how you can modify the callLanguageModelAPI
function from the previous blog posts to include the max_tokens
parameter:
function callLanguageModelAPI(givenPrompt, modelName, maxTokens) {
const apiUrl = 'https://api.openai.com/v1/chat/completions';
const apiKey = 'your-api-key-here';
const messageForAI = [{role: "user", content: givenPrompt}];
const options = {
method: 'post',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer '+apiKey
},
payload: JSON.stringify({
messages: messageForAI,
model: modelName,
max_tokens: maxTokens })
};
const response = UrlFetchApp.fetch(apiUrl, options);
const json = JSON.parse(response.getContentText());
// Access the generated message content
const generatedMessage = json['choices'][0]['message']['content'];
return generatedMessage;
}
To control the response length, simply pass the desired max_tokens
value when calling the callLanguageModelAPI
function:
const prompt = 'Summarize the key points of a long document.';
const modelName = 'gpt-3.5-turbo';
const maxTokens = 50;
const summary = callLanguageModelAPI(prompt, modelName, maxTokens);
Logger.log(summary);
Setting the max_tokens
parameter can help you generate more concise or longer content, depending on your requirements.
Managing Randomness with Temperature
The temperature
parameter in AI language models allows you to control the randomness of the generated content. A higher temperature value results in more random and creative output, while a lower value makes the output more focused and deterministic.
In Google App Script, you can set the temperature
parameter when making an API call to the AI language model. Here’s how you can modify the callLanguageModelAPI
function to include the temperature
parameter:
In Google App Script, you can set the temperature
parameter when making an API call to the AI language model. Here’s how you can modify the callLanguageModelAPI
function to include the temperature
parameter:
function callLanguageModelAPI(givenPrompt, modelName, maxTokens, temperature) {
const apiUrl = 'https://api.openai.com/v1/chat/completions';
const apiKey = 'your-api-key-here';
const messageForAI = [{role: "user", content: givenPrompt}];
const options = {
method: 'post',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer '+apiKey
},
payload: JSON.stringify({
messages: messageForAI,
model: modelName,
max_tokens: maxTokens,
temperature: temperature
})
}
// Example usage with max_tokens and temperature parameters
const prompt = 'Write a creative story about a magical forest.';
const modelName = 'gpt-3.5-turbo';
const maxTokens = 100;
const temperature = 0.8;
const story = callLanguageModelAPI(prompt, modelName, maxTokens, temperature);
Logger.log(story);
By adjusting the temperature
parameter, you can control the level of creativity and randomness in the generated content to match your specific use case.
Striking the Right Balance
Controlling response length and randomness is crucial for creating AI-driven content that meets your requirements. By fine-tuning the max_tokens
and temperature
parameters in Google App Script, you can strike the right balance between brevity, creativity, and focus in the content generated by AI language models like GPT-3.5 Turbo and GPT-4.
Here are a few tips to help you find the optimal settings for your application:
- Experiment with different values: There is no one-size-fits-all solution, so it’s essential to experiment with various parameter values to find the optimal settings for your use case.
- Consider the context: The ideal response length and randomness may vary depending on the context of your application. For example, a creative writing app may benefit from higher randomness, while a technical documentation tool may require more deterministic output.
- Test and iterate: Continuously test and refine your parameter settings based on user feedback and the quality of the generated content. This iterative process can help you enhance the overall effectiveness of your Google App Script applications.
Conclusion
Controlling response length and randomness is a critical aspect of working with AI language models in Google App Script applications. By adjusting parameters like max_tokens
and temperature
, you can tailor the generated content to suit your specific needs and requirements.
With a solid understanding of these parameters and the techniques discussed in this blog post, you can create more targeted, relevant, and engaging AI-driven content for your Google App Script projects, improving the overall user experience and the value your applications provide.