Use Case-Specific Prompt Engineering in Google App Script with AI Language Models
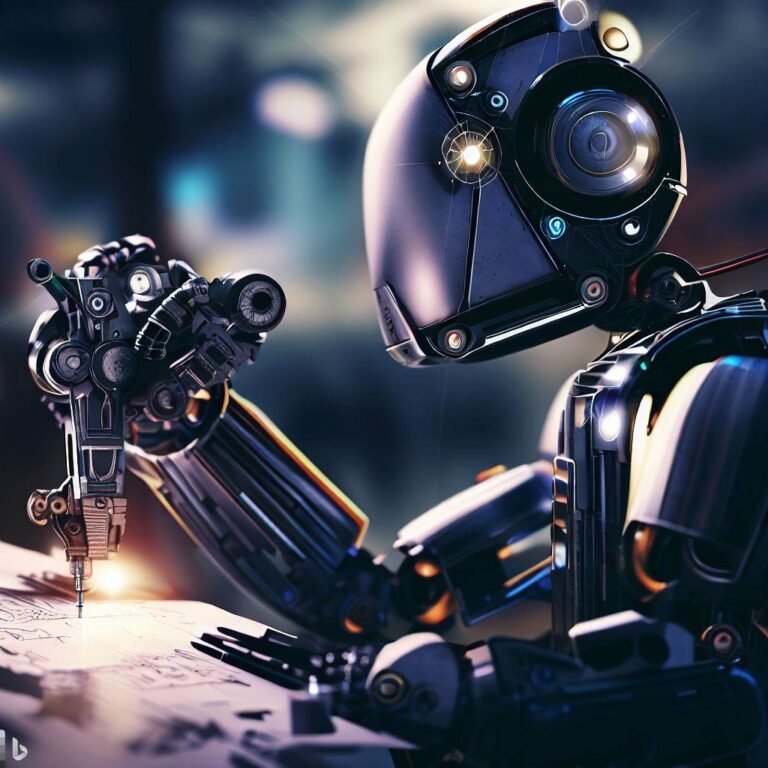
Throughout my website, following the links to any of my affiliates and making a purchase will help support my efforts to provide you great content! My current affiliate partners include ZimmWriter, LinkWhisper, Bluehost, Cloudways, Crocoblock, RankMath Pro, Parallels for Mac, AppSumo, and NeuronWriter (Lifetime Deal on AppSumo).
For tutorials on how to use these, check out my YouTube Channel!
When working with AI language models like GPT-3.5 Turbo and GPT-4 in Google App Script applications, it’s essential to tailor prompt engineering techniques to the specific use case at hand. Adapting prompt engineering strategies to the unique requirements and constraints of each use case ensures that the AI-generated content is relevant, accurate, and effective.
In this blog post, we’ll explore various use cases and provide practical guidance on how to engineer prompts for each scenario when using Google App Script with AI language models. We’ll cover common use cases, such as content generation, information extraction, and conversational agents.
Use Case 1: Content Generation
Content generation is a popular use case for AI language models, as they can produce text that is creative, engaging, and relevant to the given topic. Here are some prompt engineering tips for content generation:
Tip 1: Be Specific in Your Prompt
When generating content, provide a detailed and specific prompt to guide the AI language model. Include the topic, desired format, and any relevant context or examples.
For example:
const prompt = 'Write a concise blog post about the benefits of adopting a plant-based diet for both personal health and environmental sustainability.';
Tip 2: Control the Length of the Generated Content
You can control the length of the generated content by adjusting the max_tokens
parameter in the API call. This is useful when generating content with specific length requirements, such as social media posts or ad copy.
const maxTokens = 280; // Generate content suitable for a tweet
Use Case 2: Information Extraction
AI language models can be used to extract specific information from text, such as dates, names, or addresses. Here are some prompt engineering tips for information extraction:
Tip 1: Use a Question-Based Prompt
Frame your prompt as a question, asking the AI language model to extract the desired information from the given text.
For example:
const text = 'John Doe was born on April 15, 1990, in New York City.';
const prompt = 'From the following text: '+text+' - What is John Doe's birthdate?';
Tip 2: Test Different Prompt Structures
Experiment with different prompt structures to find the most effective way to extract the desired information. For example, you might try rephrasing the question or providing additional context.
Use Case 3: Conversational Agents
AI language models can be used to build conversational agents that can understand and respond to user inputs in a natural and engaging manner. Here are some prompt engineering tips for conversational agents:
Tip 1: Use a Conversation-Based Prompt Structure
Structure your prompt as a conversation between the user and the AI assistant, providing the user’s input and any relevant context.
For example:
const userInput = 'What are some healthy meal options for dinner?';
const prompt = 'You are a helpful assistant. User: '+userInput';
Tip 2: Maintain Conversation History
When building a multi-turn conversational agent, maintain the conversation history by including previous user inputs and AI-generated responses in the prompt.
For example, to include the conversation history in your prompt, you can use the following code:
const conversation = [
{role: 'user', content: 'What are some healthy meal options for dinner?'},
{role: 'assistant', content: 'Here are a few healthy dinner ideas: Grilled vegetables with quinoa, lentil soup with whole-grain bread, or a tofu stir-fry with brown rice.'},
{role: 'user', content: 'How do I make the lentil soup?'}
];
const prompt = conversation.map(msg => `(${msg.role}) ${msg.content}`).join('\n');
Tip 3: Experiment with Temperature Settings
To control the randomness and diversity of AI-generated responses, experiment with the temperature
when making API calls.
For example, you can set a lower temperature to generate more focused and deterministic responses:
const temperature = 0.7; // Adjust temperature to control randomness
Use Case 4: Translation and Language Tasks
AI language models can be used to perform translation and other language-related tasks. Here are some prompt engineering tips for language tasks:
Tip 1: Specify the Source and Target Languages
When crafting a prompt for translation or other language tasks, be sure to specify both the source and target languages.
For example:
const sourceText = 'Bonjour, comment ça va?';
const prompt = 'Translate the following French text to English: ' +sourceText;
Tip 2: Provide Context and Examples
For complex language tasks or when working with less common languages, provide additional context or examples to help guide the AI language model.
Conclusion
Tailoring prompt engineering strategies to each use case is crucial when working with AI language models like GPT-3.5 Turbo and GPT-4 in Google App Script applications. By following the tips and techniques shared in this blog post, you can improve the quality and relevance of AI-generated content across various use cases, from content generation and information extraction to conversational agents and language tasks.
Remember that experimentation and iteration are key aspects of prompt engineering, and refining your prompts based on your specific use case and requirements will help you achieve better results with AI language models in your Google App Script applications.