Iterative Prompt Engineering in Google App Script with AI Language Models
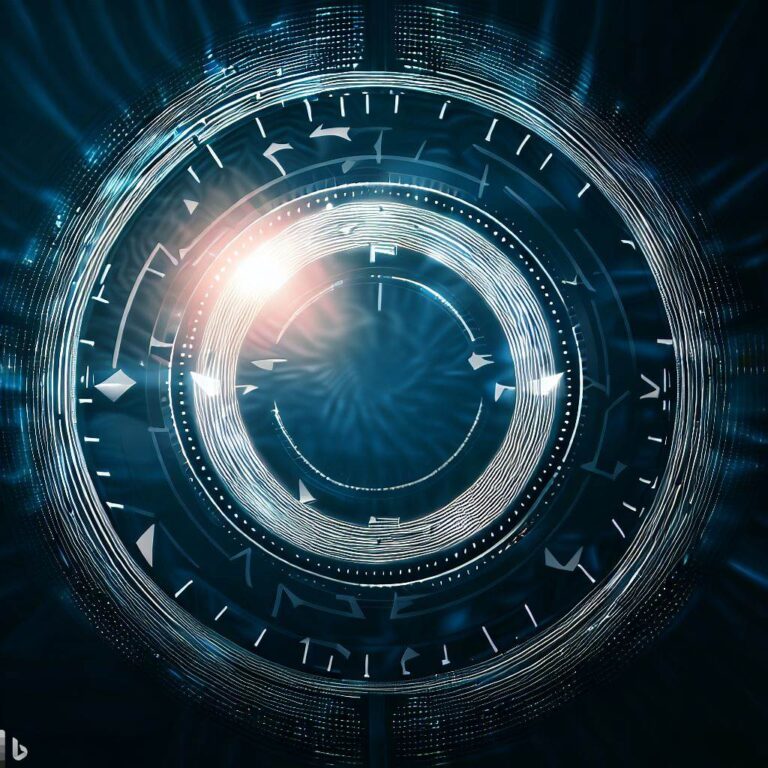
Throughout my website, following the links to any of my affiliates and making a purchase will help support my efforts to provide you great content! My current affiliate partners include ZimmWriter, LinkWhisper, Bluehost, Cloudways, Crocoblock, RankMath Pro, Parallels for Mac, AppSumo, and NeuronWriter (Lifetime Deal on AppSumo).
For tutorials on how to use these, check out my YouTube Channel!
Iterative prompt engineering is a powerful approach for optimizing the performance of AI language models, like GPT-3.5 Turbo and GPT-4, in your Google App Script applications. By refining prompts and adjusting parameters through a series of iterations, you can enhance the quality and relevance of the generated content.
In this blog post, we will explore the process of iterative prompt engineering in Google App Script, providing you with the tools and techniques to optimize AI-driven content generation in your applications.
The Iterative Prompt Engineering Process
Iterative prompt engineering involves a series of steps to gradually improve the performance of AI language models in generating content that aligns with your specific requirements. The process can be broken down into the following stages:
- Initial prompt creation: Develop an initial prompt based on your desired output and the context of your application.
- Model testing: Test the AI language model using the initial prompt and assess the quality of the generated content.
- Prompt refinement: Refine the prompt and adjust parameters based on the observed performance and any issues identified.
- Iteration: Repeat the model testing and prompt refinement steps until the desired performance and content quality are achieved.
Let’s dive deeper into each stage of this process and see how they can be applied in the context of Google App Script.
Initial Prompt Creation
The first step in iterative prompt engineering is to create an initial prompt that captures the essence of the desired output. Keep in mind the specific context of your application and the type of content you want the AI language model to generate. A well-crafted prompt should:
- Be clear and concise, providing the AI model with a clear understanding of the task.
- Incorporate relevant context to guide the AI model towards the desired output.
- Use any relevant examples or sample content to provide additional guidance, if necessary.
Here’s an example of an initial prompt for a Google App Script application that generates personalized workout routines:
const prompt = 'Create a personalized workout routine for someone who is new to strength training and wants to work out three days a week, targeting different muscle groups each day.';
Model Testing
Once you have crafted an initial prompt, the next step is to test the AI language model and evaluate the generated content. Using the callLanguageModelAPI
function from the previous blog posts, you can send the prompt to the AI model and receive a generated response.
Here’s an example of how to test the AI language model with the initial prompt:
const modelName = 'gpt-3.5-turbo';
const generatedContent = callLanguageModelAPI(prompt, modelName);
Logger.log(generatedContent);
After receiving the generated content, assess its quality and relevance based on your specific requirements. Consider whether the content is accurate, well-structured, and useful to the end-user of your application.
Prompt Refinement
Based on the results of the model testing, you may need to refine the prompt and adjust parameters to improve the performance of the AI language model. This may involve:
- Rewriting the prompt to be more specific or detailed, providing additional context or guidance.
- Adjusting the
max_tokens
parameter to control the response length, ensuring the generated content is concise or comprehensive as needed. - Modifying the
temperature
parameter to control the level of creativity and randomness in the output, making it more focused or exploratory as required.
For example, if the initial prompt produced a workout routine that lacked clear instructions, you could refine the prompt to include a specific request for step-by-step guidance:
const refinedPrompt = 'Create a personalized workout routine with step-by-step instructions for someone who is new to strength training and wants to work out three days a week, targeting different muscle groups each day.';
Iteration
The process of iterative prompt engineering involves repeating the model testing and prompt refinement steps until the AI language model generates content that meets your desired performance and quality criteria.
For example, you might run the following loop in Google App Script, which iterates over the prompt refinement process:
let iteration = 0;
let maxIterations = 5;
let prompt = refinedPrompt;
while (iteration < maxIterations) {
const generatedContent = callLanguageModelAPI(prompt, modelName);
const contentQuality = assessContentQuality(generatedContent); // Custom function to evaluate content quality
if (contentQuality > desiredQualityThreshold) {
break;
}
prompt = refinePrompt(prompt); // Custom function to refine the prompt based on feedback. Note: This is a theoretical function.
iteration++;
}
This loop calls the AI language model with the refined prompt, evaluates the quality of the generated content using a custom assessContentQuality
function, and refines the prompt if needed, using a custom refinePrompt
function.
Keep in mind that the example above is a simplified representation of the iterative process. In practice, you might need to incorporate user feedback, domain-specific knowledge, and other contextual factors to refine the prompt and evaluate content quality effectively.
Conclusion
Iterative prompt engineering is an essential technique for optimizing the performance of AI language models like GPT-3.5 Turbo and GPT-4 in Google App Script applications. By following the iterative process of initial prompt creation, model testing, prompt refinement, and iteration, you can gradually improve the quality and relevance of the generated content, tailoring it to meet the specific requirements of your use case.
With the insights and techniques shared in this blog post, you will be better equipped to harness the full potential of AI language models in your Google App Script projects, creating more effective and engaging AI-driven content for your users.