The Science Behind Neural Networks: How Language Models Like GPT-4 Are Built and Trained
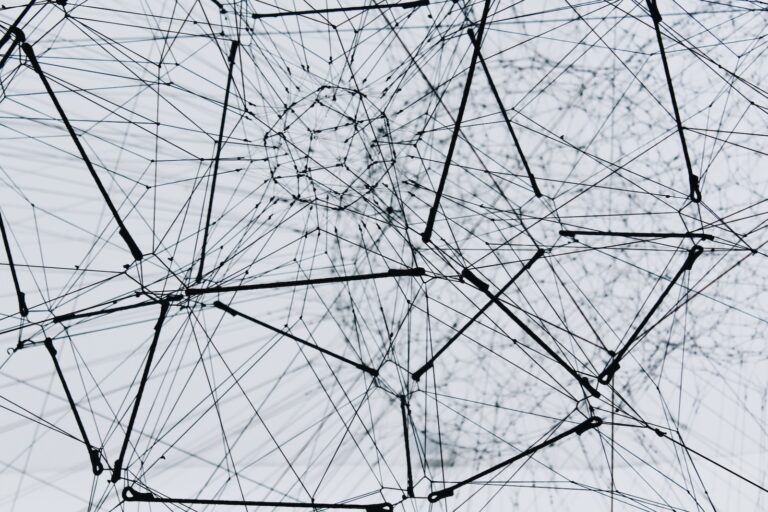
Throughout my website, following the links to any of my affiliates and making a purchase will help support my efforts to provide you great content! My current affiliate partners include ZimmWriter, LinkWhisper, Bluehost, Cloudways, Crocoblock, RankMath Pro, Parallels for Mac, AppSumo, and NeuronWriter (Lifetime Deal on AppSumo).
For tutorials on how to use these, check out my YouTube Channel!
Artificial Intelligence (AI) has come a long way, and at the forefront of these advances are neural networks. In this post, we’ll dive into the science behind neural networks and explore how state-of-the-art language models like GPT-4 are built and trained. We’ll cover the basics of deep learning, the architecture of neural networks, and the training process for language models.
Introduction to Deep Learning
Deep learning is a subfield of machine learning that focuses on using artificial neural networks to model and solve complex problems. Neural networks are inspired by the structure and function of the human brain and consist of interconnected layers of nodes or neurons.
The Neuron: The Building Block of Neural Networks
A neuron in a neural network takes in one or more inputs, applies a weighted sum, and passes the result through an activation function to produce an output. The activation function is a non-linear function that determines the output of the neuron based on the input it receives.
Here’s a simple Google Apps Script code snippet that demonstrates a basic neuron:
function neuron(inputs, weights, bias, activationFunction) { var weightedSum = 0;
for (var i = 0; i < inputs.length; i++) {
weightedSum += inputs[i] * weights[i];
}
weightedSum += bias;
return activationFunction(weightedSum);
}
function sigmoid(x) {
return 1 / (1 + Math.exp(-x));
}
function main() {
var inputs = [0.5, 0.7, 0.2];
var weights = [0.1, 0.8, 0.5];
var bias = 0.3;
var output = neuron(inputs, weights, bias, sigmoid);
Logger.log(output);
}
main();
This Google Apps Script code snippet demonstrates a simple neuron implementation. The neuron function takes inputs, weights, bias, and an activation function as arguments, and then computes the output of the neuron. The main function initializes the inputs, weights, and bias, and calls the neuron function with the sigmoid activation function.
Layers in a Neural Network
Neural networks are typically composed of several layers, which can be divided into three categories:
- Input layer: This is where the network receives input data.
- Hidden layers: These layers are responsible for processing and transforming the input data. They consist of multiple neurons, and there can be one or more hidden layers in a network.
- Output layer: This layer produces the final output or prediction of the network.
Training a Neural Network
Training a neural network involves adjusting the weights and biases of the neurons to minimize the difference between the predicted output and the actual output, a process known as optimization. This is achieved through a process called backpropagation and an optimization algorithm, such as gradient descent.
Loss Function
The loss function is used to quantify the difference between the predicted output and the actual output. The goal during training is to minimize the loss. A common loss function for classification tasks is the cross-entropy loss.
Here’s a simple Google Apps Script implementation of cross-entropy loss:
function crossEntropyLoss(yTrue, yPred) {
var loss = 0;
for (var i = 0; i < yTrue.length; i++) {
loss += yTrue[i] * Math.log(yPred[i]);
}
return -loss;
}
function main() {
var yTrue = [1, 0, 0];
var yPred = [0.7, 0.2, 0.1];
var loss = crossEntropyLoss(yTrue, yPred);
Logger.log(loss);
}
main();
This Google Apps Script code snippet demonstrates a simple implementation of the cross-entropy loss function. The crossEntropyLoss
function takes two arrays, yTrue
and yPred
, which represent the actual output and the predicted output, respectively. The function computes the loss using the cross-entropy formula, and the main function initializes the arrays and calls the crossEntropyLoss
function to calculate the loss.
Backpropagation is an algorithm that calculates the gradient of the loss function with respect to each weight and bias by applying the chain rule from calculus. The gradients are then used by the optimization algorithm to update the weights and biases.
Optimization Algorithm: Gradient Descent
Gradient descent is an optimization algorithm that adjusts the weights and biases of the network to minimize the loss function. It does this by taking steps in the direction of the negative gradient, which leads to the lowest point of the loss function.
Here’s a simple Google Apps Script implementation of gradient descent for a single weight:
function gradientDescent(weight, gradient, learningRate) {
return weight - learningRate * gradient;
}
function main() {
var weight = 0.5;
var gradient = 0.8;
var learningRate = 0.01;
var newWeight = gradientDescent(weight, gradient, learningRate);
Logger.log(newWeight);
}
main();
This Google Apps Script code snippet demonstrates a simple implementation of the gradient descent algorithm for a single weight. The gradientDescent
function takes three arguments: the current weight, the gradient, and the learning rate. It updates the weight by subtracting the product of the learning rate and the gradient from the current weight. The main function initializes the weight, gradient, and learning rate, then calls the gradientDescent
function to update the weight.
Building and Training Language Models Like GPT-4
Now that we understand the basics of neural networks and the training process, let’s discuss how language models like GPT-4 are built and trained.
Transformers: The Core of GPT-4
GPT-4, like its predecessors GPT-3 and GPT-2, is based on the Transformer architecture. Transformers are a type of neural network that are particularly well-suited for natural language processing (NLP) tasks because of their ability to model long-range dependencies and handle variable-length input sequences.
The Transformer architecture consists of an encoder and a decoder, each composed of multiple self-attention layers and feed-forward layers. In the case of GPT-4, which is an autoregressive language model, only the decoder is used.
Tokenization and Preprocessing
To train a language model like GPT-4, the text data needs to be tokenized and preprocessed. Tokenization involves splitting the text into smaller units called tokens, which can be words, subwords, or characters, depending on the chosen tokenization strategy. The tokens are then mapped to unique IDs that can be fed into the model.
Fine-Tuning the Model
Once the data is preprocessed, GPT-4 can be fine-tuned on a specific task or dataset. Fine-tuning involves updating the pretrained model’s weights and biases with a smaller dataset and a more specific task, allowing the model to generalize its knowledge and adapt to new tasks more effectively.
// Pseudo code for fine-tuning a language model like GPT-4 in Google Apps Script
function loadPretrainedModel() {
// Load the pretrained GPT-4 model
}
function loadTokenizer() {
// Load the tokenizer for GPT-4
}
function preprocessData(rawData, tokenizer) {
// Preprocess the raw data using the tokenizer
}
function trainModel(pretrainedModel, trainData) {
// Fine-tune the pretrained model on the given dataset
}
function main() {
var pretrainedModel = loadPretrainedModel();
var tokenizer = loadTokenizer();
// Prepare the data
var raw_data = "your_raw_data";
var trainData = preprocessData(raw_data, tokenizer);
// Fine-tune the model
var fineTunedModel = trainModel(pretrainedModel, trainData);
}
main();
Please note that this is a high-level representation of the fine-tuning process in Google Apps Script. In practice, fine-tuning a complex model like GPT-4 would require a more powerful computing environment and specialized libraries that are not available in Google Apps Script. This example is provided to demonstrate the high-level steps involved in the fine-tuning process.
Although I haven’t yet tested this code, I have found that it may be used for fine-tuning using Python.
#Python Code
# Pseudo code for fine-tuning a language model like GPT-4
pretrained_model = load_pretrained_model()
tokenizer = load_tokenizer()
# Prepare the data
train_data = preprocess_data(raw_data, tokenizer)
# Fine-tune the model
fine_tuned_model = train_model(pretrained_model, train_data)
Conclusion
In this post, we have explored the science behind neural networks, focusing on how language models like GPT-4 are built and trained. We’ve covered the basics of deep learning, neural network architecture, and the training process. As AI continues to advance, language models like GPT-4 will undoubtedly play a crucial role in transforming the way we work and live. By understanding the underlying concepts and technology, we can better harness the power of these cutting-edge AI tools.