API Keys and You: A Step-by-Step Guide to Accessing and Utilizing OpenAI's Services
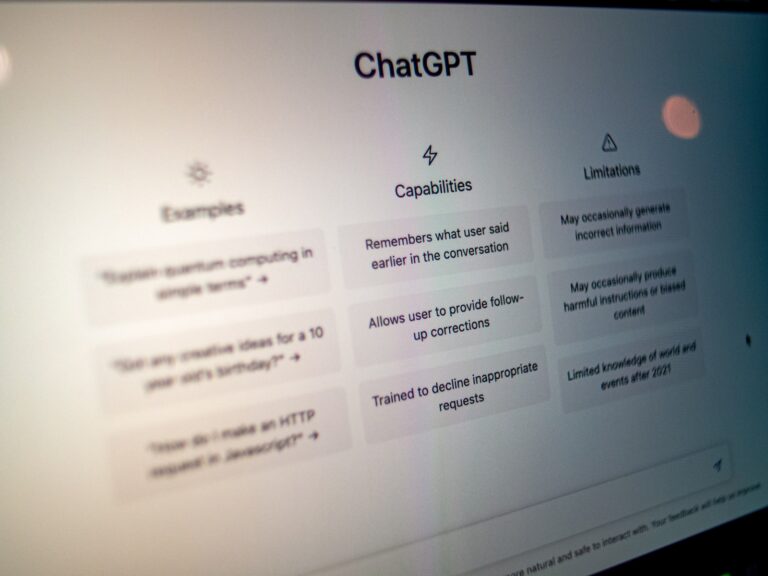
Throughout my website, following the links to any of my affiliates and making a purchase will help support my efforts to provide you great content! My current affiliate partners include ZimmWriter, LinkWhisper, Bluehost, Cloudways, Crocoblock, RankMath Pro, Parallels for Mac, AppSumo, and NeuronWriter (Lifetime Deal on AppSumo).
For tutorials on how to use these, check out my YouTube Channel!
1. Introduction
OpenAI offers a variety of powerful AI services, such as GPT-4, ChatGPT, and DALL-E-2, that can be accessed through its API. To use these services, you need to have an API key. In this guide, we’ll walk you through the process of signing up for OpenAI, obtaining your API key, and using it in your Google Apps Script projects. Along the way, we’ll provide plenty of code examples to help you get started.
2. Signing Up for OpenAI
Before you can access OpenAI’s API, you need to create an account on their website. Follow these steps to sign up:
- Go to the OpenAI website.
- Click “Get started for free” or “Sign up” in the upper right corner of the homepage.
- Fill out the required fields, agree to the terms and conditions, and click “Create account.”
- You’ll receive a verification email. Click the link in the email to confirm your email address and activate your account.
After signing up, you can access the OpenAI dashboard, which provides an overview of your API usage and other information related to your account.
3. Obtaining Your API Key
Once you’ve created an OpenAI account, you can obtain your API key by following these steps:
- Log in to the OpenAI dashboard with your account credentials.
- In the left sidebar, click on “API keys.”
- Click on the “Create API key” button.
- Give your API key a name and optionally set usage limits to prevent accidental overuse.
- Click “Create” to generate your API key. Make sure to copy your API key and store it in a safe place, as you won’t be able to see it again.
Your API key serves as your unique identifier when making API calls to OpenAI’s services, so it’s essential to keep it secure and avoid sharing it with others.
4. Using Your API Key in Google Apps Script
To use your OpenAI API key in Google Apps Script, follow these steps:
- Create a new Google Apps Script project in your Google Drive. Click “New,” then “More,” and select “Google Apps Script.”
- Now, on the left hand side of the screen, click “Project Settings” > “Script Properties” > “Add Script Property.”
- Click the “+” button to add a new property.
- Set the property name as “openai_api_key” and enter your API key as the value. Click “Save.”
By storing your API key as a script property, you can easily access and use it throughout your project without including it directly in your code, providing an additional layer of security.
5. Creating a Function to Call OpenAI’s API
Now that your API key is stored in your Google Apps Script project, you can create a function to call OpenAI’s API. In this example, we’ll create a function that sends a prompt to GPT-4 and returns the generated response.
// Import the URL Fetch Service const UrlFetchApp = require('UrlFetchApp'); function callOpenAIAPI(prompt) { const apiKey = PropertiesService.getScriptProperties().getProperty('openai_api_key'); const url = 'https://api.openai.com/v1/chat/completions
'; const options = { method: 'POST', headers: { 'Content-Type': 'application/json', 'Authorization': 'Bearer ' + apiKey }, payload: JSON.stringify({ prompt: [{role: "user", content: prompt}], max_tokens: 1000, n: 1, stop: null, temperature: 1.0, top_p: 1.0 }) }; const response = UrlFetchApp.fetch(url, options); const jsonResponse = JSON.parse(response.getContentText()); return
json['choices'][0]['message']['content']}
6. Example: Using GPT-4 with Your API Key
With the callOpenAIAPI()
function defined, you can now use GPT-4 in your Google Apps Script project. Let’s create a simple example that sends a prompt to GPT-4 and logs the generated response.
function testGPT4() {
const prompt = 'Write a short introduction paragraph about artificial intelligence:';
const response = callOpenAIAPI(prompt);
Logger.log(response);
}
Run the testGPT4()
function in your script editor, and check the logs (View > Logs) to see the generated response from GPT-4.
7. Best Practices for Managing API Keys
To ensure the security and proper usage of your API key, follow these best practices:
- Never share your API key with others or include it directly in your code. Instead, store it in a secure location, such as script properties or environment variables.
- Set usage limits for your API key to prevent accidental overuse and control your costs.
- Regularly monitor your API usage to detect any unusual activity and make sure you stay within your plan limits.
- Rotate your API key periodically to minimize the risk of unauthorized access. If you suspect your key has been compromised, revoke it immediately and generate a new one.
8. Conclusion
In this guide, we walked you through the process of obtaining and using an OpenAI API key in your Google Apps Script projects. We showed you how to store your API key securely, create a function to call OpenAI’s API, and use GPT-4 with your API key. By following these steps and best practices, you can unlock the power of OpenAI’s services and incorporate them into your own projects and workflows.