Part 9: Best Practices and Common Pitfalls
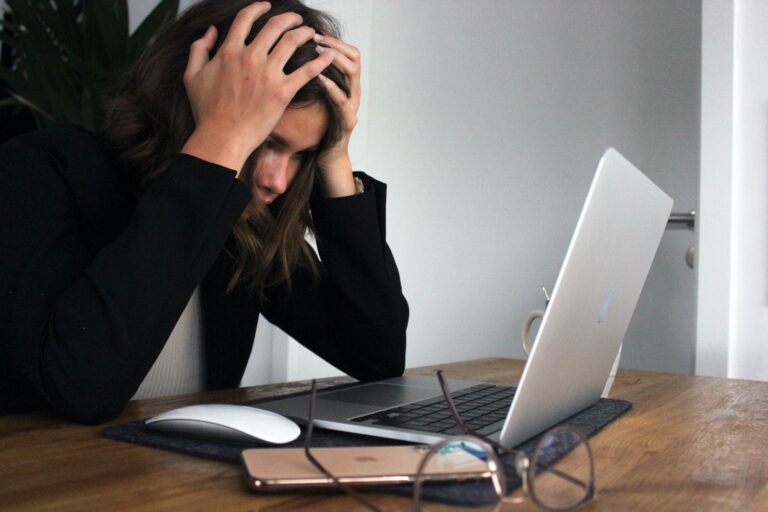
Throughout my website, following the links to any of my affiliates and making a purchase will help support my efforts to provide you great content! My current affiliate partners include ZimmWriter, LinkWhisper, Bluehost, Cloudways, Crocoblock, RankMath Pro, Parallels for Mac, AppSumo, and NeuronWriter (Lifetime Deal on AppSumo).
For tutorials on how to use these, check out my YouTube Channel!
In this blog post, we’ll cover best practices and common pitfalls when working with Google App Script, particularly in the context of Google Sheets automation. By following these guidelines, you can write more efficient and maintainable code and avoid common mistakes that could lead to unexpected behavior or performance issues.
Best Practices
1. Use Comments to Explain Your Code
Using comments to explain your code helps both you and others understand the purpose and functionality of the script. This is particularly important when working with complex code or when collaborating with others. To add a comment in Google App Script, use the double forward-slash (//
) for single-line comments or /* */
for multi-line comments:
// This is a single-line comment
/*
This is a
multi-line comment
*/
2. Break Your Code into Functions
Breaking your code into smaller, modular functions improves readability and makes it easier to maintain and troubleshoot. Functions should generally have a single responsibility, and you can reuse them in different parts of your script.
function getActiveSheet() {
return SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
}
function getLastRow() {
return getActiveSheet().getLastRow();
}
3. Use Descriptive Variable and Function Names
Using descriptive variable and function names makes your code easier to understand. Choose names that reflect the purpose or content of the variable, and use camelCase notation for readability.
// Good example
function getLastRow() {
let sheet = getActiveSheet();
return sheet.getLastRow();
}
// Bad example
function lr() {
let s = gas();
return s.lr();
}
4. Minimize API Calls
Minimizing API calls helps reduce the execution time of your script and prevent hitting usage limits. Instead of making repeated API calls to fetch the same data, store the data in a variable and reuse it throughout your script.
// Good example
function getSheetData() {
let sheet = getActiveSheet();
let lastRow = sheet.getLastRow();
let dataRange = sheet.getRange(1, 1, lastRow, 2);
return dataRange.getValues();
}
// Bad example
function getCellValue(row, column) {
let sheet = getActiveSheet();
return sheet.getRange(row, column).getValue();
}
5. Use Error Handling
Using error handling helps you manage unexpected issues that might occur during the execution of your script. Use try-catch
blocks to catch and handle errors gracefully.
function fetchDataFromAPI(apiUrl) {
try {
let response = UrlFetchApp.fetch(apiUrl);
return JSON.parse(response.getContentText());
} catch (error) {
console.error('Error fetching data from '+apiUrl+': '+error);
return null;
}
}
Common Pitfalls
1. Incorrectly Accessing Spreadsheet Data
When working with Google Sheets, ensure you are correctly accessing the sheet, range, and cell data. A common mistake is using getValue()
or setValue()
on a range object instead of a cell object.
// Correct usage
let cellValue = sheet.getRange(1, 1).getValue();
sheet.getRange(1, 2).setValue(cellValue);
// Incorrect usage
let cellValue = sheet.getRange(1, 1).getValue; // Missing parentheses
sheet.getRange(1, 2).setValue = cellValue; // Incorrect assignment
2. Not Handling Empty or Invalid Data
Make sure your script can handle cases where input data
is empty or invalid. Validate your data and provide appropriate error messages or default values when necessary.
function processInputData(input) {
if (!input || input === "") {
console.error("Input data is empty or invalid");
return;
}
// Process the input data here
}
3. Ignoring Quotas and Execution Time Limits
Be aware of the quotas and limits imposed by Google App Script. Scripts exceeding these limits may result in errors or unexpected behavior. Design your scripts to stay within these limits and handle any errors that might occur when reaching them.
4. Failing to Test and Debug
Always test your scripts and debug any issues that may arise. You can use the built-in debugging tools in the Google App Script editor, including breakpoints, watch expressions, and the Logger. Additionally, make use of console.log()
statements to monitor the progress and state of your script during execution.
function processSheetData() {
let data = getSheetData();
console.log("Fetched sheet data:", data);
data.forEach((row, index) => {
console.log('Processing row '+(index + 1)+', row);
// Process each row here
});
}
5. Forgetting to Save Your Work
Make sure you save your work regularly. The Google App Script editor does not auto-save your changes, so be sure to click the “Save” button or use the keyboard shortcut (Ctrl + S or Cmd + S) frequently to avoid losing your progress.
Conclusion
By following the best practices and avoiding common pitfalls mentioned in this blog post, you can write more efficient, maintainable, and reliable Google App Script code. As you continue to work with Google Sheets and Google App Scripts, keep refining your skills and stay up to date with new features and improvements in the platform.
In the next and final blog post of this series, we’ll explore other applications for Google App Scripts beyond Google Sheets, expanding your automation capabilities within the Google Workspace.