Part 7: Using OpenAI's GPT-3.5-turbo or GPT-4 for Content Generation in Google Sheets
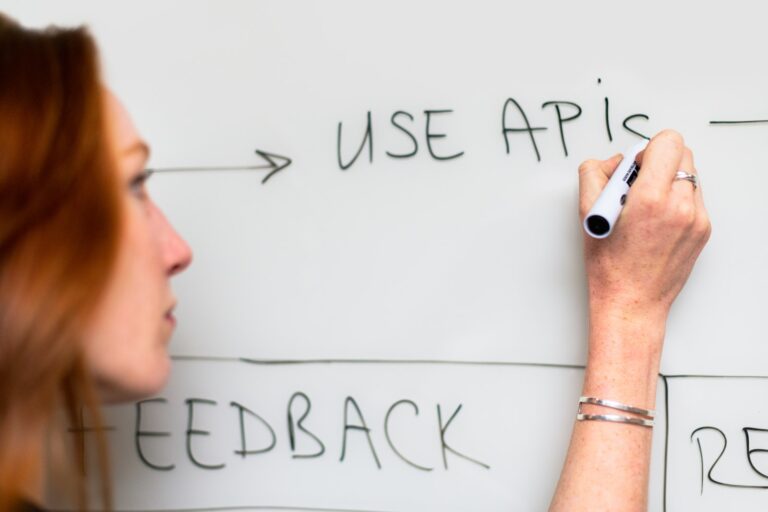
Throughout my website, following the links to any of my affiliates and making a purchase will help support my efforts to provide you great content! My current affiliate partners include ZimmWriter, LinkWhisper, Bluehost, Cloudways, Crocoblock, RankMath Pro, Parallels for Mac, AppSumo, and NeuronWriter (Lifetime Deal on AppSumo).
For tutorials on how to use these, check out my YouTube Channel!
In this blog post, we will demonstrate how to integrate OpenAI’s powerful GPT-3.5-turbo language model with Google Sheets using Google App Scripts. This will allow you to generate content directly within your spreadsheet, opening up numerous possibilities for content creation and data analysis.
Introduction to OpenAI’s GPT-3.5-turbo
GPT-3.5-turbo is one of OpenAI’s most advanced language models, capable of understanding and generating human-like text. It can be used for various tasks, such as content generation, translation, summarization, question-answering, and more.
To access the GPT-3.5-turbo API, you will need an API key from OpenAI. Visit the OpenAI website to learn more about their API and sign up for an API key.
Setting up GPT-3.5-turbo API Calls in Google App Script
To interact with the GPT-3.5-turbo API in Google App Script, you will need to make HTTP POST requests using the UrlFetchApp
class.
First, create a new script in your Google Sheets script editor by clicking “Extensions” in the top menu, then “Apps Script.
Before we start writing code, let’s add your OpenAI API key as a script property. This way, you won’t accidentally share your API key when sharing your code.
On the left hand side, select “Project Settings” > “Script Properties” > “Add Script Property” and add a new property with the key “OPENAI_API_KEY” and your OpenAI API key as the value.
Now, create a custom function in the script editor to call the GPT-3.5-turbo API:
function callGpt35Turbo(prompt) {
let apiKey = PropertiesService.getScriptProperties().getProperty('OPENAI_API_KEY');
let apiUrl = 'https://api.openai.com/v1/chat/completions';
var messageForAI = [{role: "user", content: prompt}]
let payload = {
'model': model,
'messages': messageForAI,
'max_tokens': 1000,
'temperature': 0.7
};
let options = {
'method': 'post',
'contentType': 'application/json',
'headers': {
'Authorization': `Bearer` + apiKey
},
'payload': JSON.stringify(payload)
};
let response = UrlFetchApp.fetch(apiUrl, options);
let responseData = JSON.parse(response.getContentText());
return responseData['choices'][0]['message']['content'];
}
This function takes a prompt
as input and sends a POST request to the GPT-3.5-turbo API with the specified prompt
and some default settings for max_tokens
and temperature
. The function returns the generated text from the API response.
Generating Content with GPT-3.5-turbo in Google Sheets
With the callGpt35Turbo
function in place, you can now generate content using GPT-3.5-turbo directly in your Google Sheets.
Create another custom function that takes a cell value as input, calls the GPT-3.5-turbo API with the input value, and writes the generated content to another cell:
function generateContentFromCell() {
let sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
let inputCell = sheet.getRange('A1');
let outputCell = sheet.getRange('B1');
let prompt = inputCell.getValue();
let generatedContent = callGpt35Turbo(prompt);
output.setValue(generatedContent);}
This generateContentFromCell
function reads the value from cell A1, uses it as a prompt for GPT-3.5-turbo, and writes the generated content to cell B1.
To test the generateContentFromCell
function, write a prompt in cell A1 of your Google Sheet (e.g., “What are the benefits of exercise?”) and then run the generateContentFromCell
function in the script editor. You should see the generated content in cell B1.
Creating a Custom Function for GPT-3.5-turbo in Google Sheets
To make it even more convenient to use GPT-3.5-turbo in your Google Sheets, you can create a custom function that can be called directly in your spreadsheet.
Create a new custom function called GPT3_GENERATE
:
function GPT3_GENERATE(prompt) {
return callGpt35Turbo(prompt);
}
Now, you can use the GPT3_GENERATE
function in your Google Sheets just like any other built-in function. For example, you can write =GPT3_GENERATE("What are the benefits of exercise?")
in a cell, and the generated content will appear in the same cell.
Note that using the GPT-3.5-turbo API consumes tokens, and each API call may have costs associated with it, depending on your OpenAI subscription plan. So be cautious about the number of requests you make and the size of the content generated.
Examples of GPT-3.5-turbo Applications in Google Sheets
Here are some ideas of how you can leverage GPT-3.5-turbo in your Google Sheets:
- Content generation: Automatically generate article summaries, product descriptions, social media posts, or marketing copy based on your input prompts.
- Data analysis: Use GPT-3.5-turbo to analyze spreadsheet data and generate human-readable insights, trends, or summaries. Be careful how much you trust this… Sometimes it likes to hallucinate.
- Translation: Translate text between languages by providing prompts like “Translate the following English text to French: {text}”.
- Code generation: Generate code snippets in various programming languages based on your prompts.
Conclusion
By integrating OpenAI’s GPT-3.5-turbo API with Google Sheets using Google App Scripts, you can unlock powerful content generation capabilities directly within your spreadsheets. This can save time, improve your workflow, and open up new possibilities for data analysis and content creation.
In the next blog post, we’ll explore how to direct GPT-3.5-turbo responses to specific cells in Google Sheets using Google App Scripts.